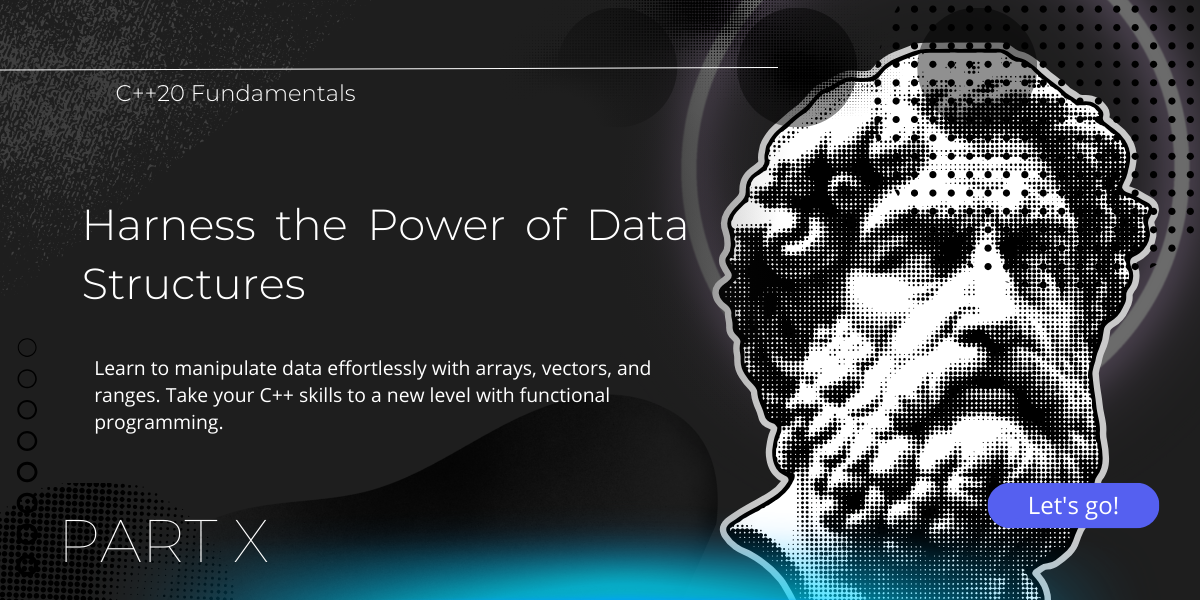
Course C++ Part 10: Arrays, Vectors, Ranges, and Functional-Style Programming
Course Description
This lesson covers the use of arrays, vectors, and ranges, introducing functional programming techniques for more elegant C++ code.
Boost your C++ programming efficiency by mastering arrays, vectors, ranges, and functional-style programming! This course explores the fundamentals of arrays and std::vector, helping you manage dynamic data structures with ease. You'll dive into C++ ranges, a modern approach to working with sequences that enhances code readability and safety. Additionally, you'll learn how to apply functional-style programming concepts, leveraging higher-order functions, lambda expressions, and algorithms to write cleaner and more expressive C++ code. Whether you're optimizing performance or improving code maintainability, these techniques will elevate your C++ skills to a new level!
Course Curriculum
- 001. Lesson 10 Overview--OOP Inheritance and Runtime Polymorphism
- 002. Base Classes and Derived Classes
- 003. Relationship between Base and Derived Classes
- 004. Creating and Using a SalariedEmployee Class
- 005. Creating a SalariedEmployee-SalariedCommissionEmployee Inheritance Hierarchy
- 006. Constructors and Destructors in Derived Classes
- 007. Relationship Among Objects in an Inheritance Hierarchy
- 008. Invoking Base-Class Functions from Derived-Class Objects
- 009. Aiming Derived-Class Pointers at Base-Class Objects
- 010. Derived-Class Member-Function Calls via Base-Class Pointers
- 011. virtual Funtions
- 012. Do Not Call virtual Functions from Constructors and Destructors
- 013. virtual Destructors
- 014. final Member Functions and Classes
- 015. Abstract Classes and Pure virtual Functions
- 016. Declaring a Pure Virtual Function
- 017. Case Study Payroll System Using Runtime Polymorphism
- 018. Creating Abstract Base-Class Employee
- 019. Creating Concrete Derived-Class SalariedEmployee
- 020. Creating Concrete Derived-Class CommissionEmployee
- 021. Demonstrating Runtime Polymorphic Processing
- 022. Runtime Polymorphism, Virtual Functions and Dynamic Binding Under the Hood
- 023. Non-Virtual Interface (NVI) Idiom
- 024. Refactoring Class Employee for the NVI Idiom
- 025. Updated Class SalariedEmployee
- 026. Updated Class CommissionEmployee
- 027. Runtime Polymorphism with the Employee Hierarchy Using NVI
- 028. 10.12 Program to an Interface, Not an Implementation
- 029. Rethinking the Employee Hierarchy--CompensationModel Interface
- 030. Class Employee
- 031. CompensationModel Implementations
- 032. Testing the New Hierarchy
- 033. Runtime Polymorphism with stdvariant and stdvisit
- 034. Runtime Polymorphism with stdvariant and stdvisit--Compensation Model Salaried
- 035. Runtime Polymorphism with stdvariant and stdvisit--Compensation Model Commission
- 036. Runtime Polymorphism with stdvariant and stdvisit--Employee Class Definition
- 037. Runtime Polymorphism with stdvariant and stdvisit--Testing Runtime Polymorphism with stdvariant and stdvisit
- 038. Multiple Inheritance
- 039. Multiple Inheritance Example
- 040. Diamond Inheritance
- 041. Eliminating Duplicate Subobjects with virtual Base-Class Inheritance
- 042. protected Class Members
- 043. public, protected and private Inheritance