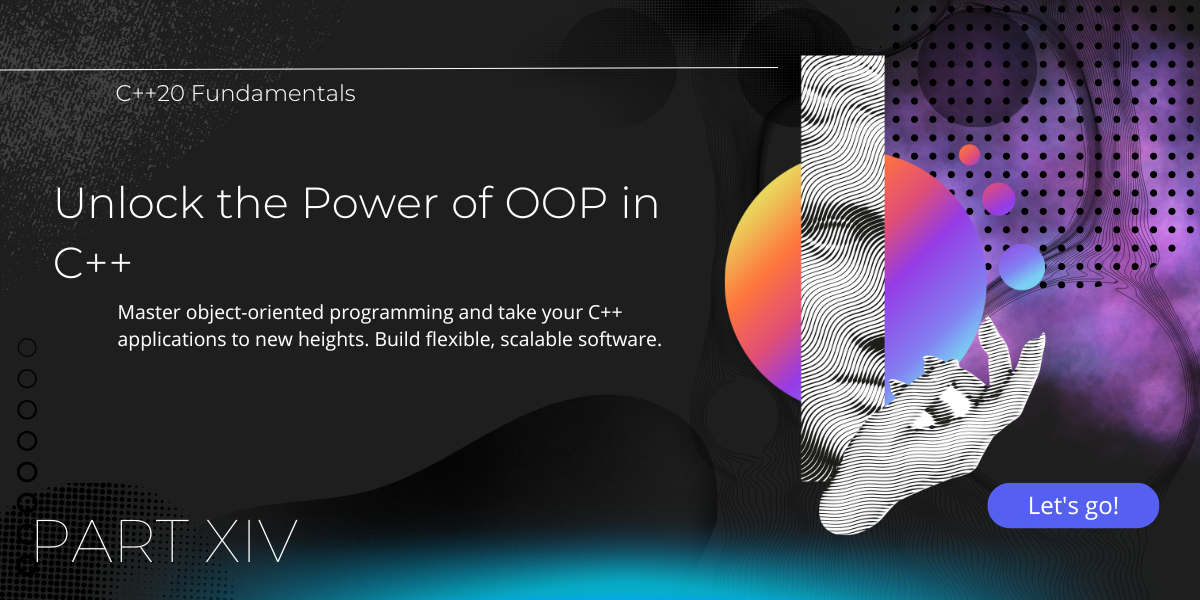
Course C++ Part 14: Object-Oriented Programming
Course Description
This course covers the fundamentals of object-oriented programming in C++, including classes, inheritance, polymorphism, and encapsulation.
Master the principles of Object-Oriented Programming (OOP) in C++ and build scalable, maintainable applications with confidence! This course covers essential OOP concepts such as encapsulation, inheritance, polymorphism, and abstraction, helping you structure your code efficiently. Learn how to design reusable classes, manage dynamic memory, and implement best practices for object-oriented development. Whether you're a beginner or looking to refine your skills, this course will provide the foundation needed to write clean, modular, and high-performance C++ applications.
Course Curriculum
- 001. Lesson 14 Overview--Standard Library Algorithms
- 002. Algorithm Requirements C++20 Concepts
- 003. Lambdas and Algorithms
- 004. Lambdas and Algorithms Part 2
- 005. fill, fill n, generate and generate n
- 006. equal, mismatch and lexicographical compare
- 007. remove, remove if, remove copy and remove copy if
- 008. replace, replace if, replace copy and replace copy if
- 009. Shuffling, Counting, and Minimum and Maximum Element Algorithms
- 010. Searching and Sorting Algorithms
- 011. swap, iter swap and swap ranges
- 012. copy backward, merge, unique, reverse, copy if and copy n
- 013. inplace merge, unique copy and reverse copy
- 014. Set Operations
- 015. lower bound, upper bound and equal range
- 016. min, max and minmax
- 017. Algorithms gcd, lcm, iota, reduce and partial sum from Header numeric
- 018. Heapsort and Priority Queues
- 019. Function Objects (Functors)
- 020. Projections
- 021. C++20 Views and Functional-Style Programming
- 022. Range Adaptors
- 023. Working with Range Adaptors and Views
- 024. A Look Ahead to C++23 Ranges