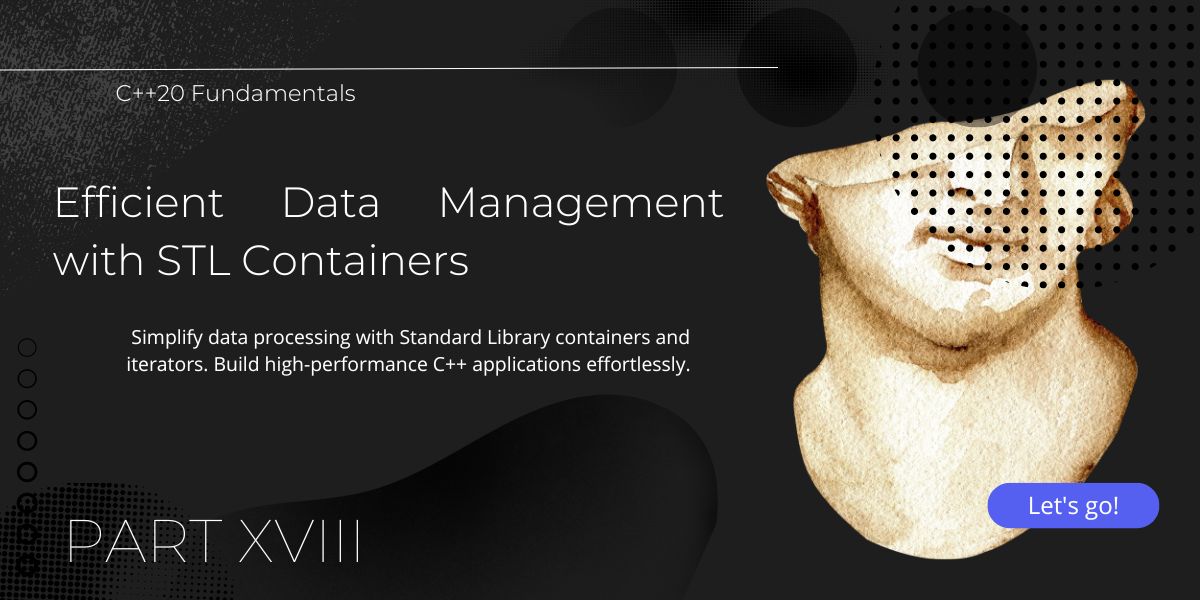
Course C++ Part 18: Standard Library Algorithms
Course Description
This course introduces you to C++ Standard Library algorithms, showing how to use them to process data and implement common programming patterns more efficiently.
Course Curriculum
- 001. Lesson 18 Overview—C++20 Coroutines
- 002. Introduction to Coroutines
- 003. Coroutine Support Libraries
- 004. Installing the concurrencpp and generator Libraries—concurrencpp Library
- 005. Installing the concurrencpp Library Using Visual Studio and vcpkg
- 006. Installing the concurrencpp Library Using Docker or Linux and vcpkg
- 007. Installing the concurrencpp and generator Libraries—generator Library
- 008. Creating a Generator Coroutine with co yield and the generator Library
- 009. Creating a Generator Coroutine with co yield and the generator Library—Diagram Showing the Flow of Control for a Generator Coroutine
- 010. Launching Tasks with concurrencpp
- 011. Launching Tasks with concurrencpp – concurrencppruntime
- 012. Launching Tasks with concurrencpp – concurrencppthread pool executor
- 013. Launching Tasks with concurrencpp – concurrencpptask and concurrencppresult
- 014. Launching Tasks with concurrencpp – Example
- 015. Launching Tasks with concurrencpp – Summary of concurrencpp Executors
- 016. Creating a Coroutine with co await and co return—Overview of This Example
- 017. Creating a Coroutine with co await and co return—Discussing the Code
- 018. Creating a Coroutine with co await and co return—Flow of Control for the Sorting Coroutine Example
- 019. Low-Level Coroutines Concepts—Resources
- 020. Low-Level Coroutines Concepts—Coroutine Restrictions
- 021. Low-Level Coroutines Concepts—Promise Object
- 022. Low-Level Coroutines Concepts—Coroutine State
- 023. Low-Level Coroutines Concepts—Coroutine Handle
- 024. Low-Level Coroutines Concepts—Awaitable Objects