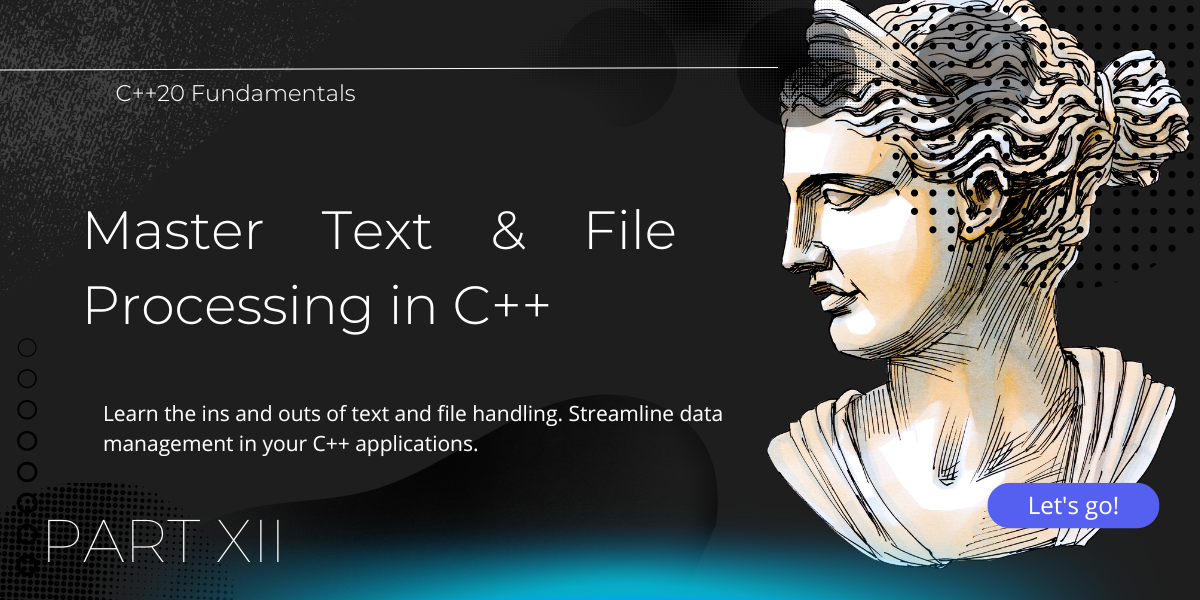
Course C++ Part 12: Strings, string_views, Text Files, CSV Files, and Regex
Course Description
This lesson focuses on working with text and file input/output in C++, including handling strings, CSV files, and using regular expressions.
Enhance your text processing skills in C++ by mastering strings, string_view, text files, CSV handling, and regular expressions! This course dives deep into efficient string manipulation techniques, exploring both std::string and std::string_view for optimal performance. You'll learn how to read, write, and parse text and CSV files, making data handling seamless. Additionally, we cover regex (regular expressions) for powerful text search, validation, and transformation. Whether you're developing data-driven applications or working with file processing, these essential techniques will take your C++ programming to the next level!
Course Curriculum
- 001. Lesson 12 Overview--Exceptions and a Look Forward to Contracts
- 002. Exception-Handling Flow of Control
- 003. Defining a catch Handler for DivideByZeroExceptions
- 004. Exception Safety Guarantees and noexcept
- 005. Rethrowing an Exception
- 006. Stack Unwinding and Uncaught Exceptions
- 007. When to Use Exception Handling
- 008. assert Macro
- 009. Failing Fast
- 010. Constructors, Destructors and Exception Handling
- 011. Throwing Exceptions from Constructors
- 012. Catching Exceptions in Constructors via Function try Blocks
- 013. Exceptions and Destructors Revisiting noexcept(false)
- 014. Processing new Failures
- 015. new Throwing bad alloc on Failure
- 016. new Returning nullptr on Failure
- 017. Handling new Failures Using Function set new handler
- 018. Standard Library Exception Hierarchy
- 019. C++’s Alternative to the finally Block Resource Acquisition Is Initialization (RAII)
- 020. Some Libraries Support Both Exceptions and Error Codes
- 021. Logging
- 022. Looking Ahead to Contracts
- 023. Looking Ahead to Contracts--Contracts Attributes and Contract Levels
- 024. Looking Ahead to Contracts--Specifying Preconditions, Postconditions and Assertions
- 025. Looking Ahead to Contracts--Early-Access Implementation
- 026. Looking Ahead to Contracts--Early-Access Implementation
- 027. Looking Ahead to Contracts--Early-Access Implementation