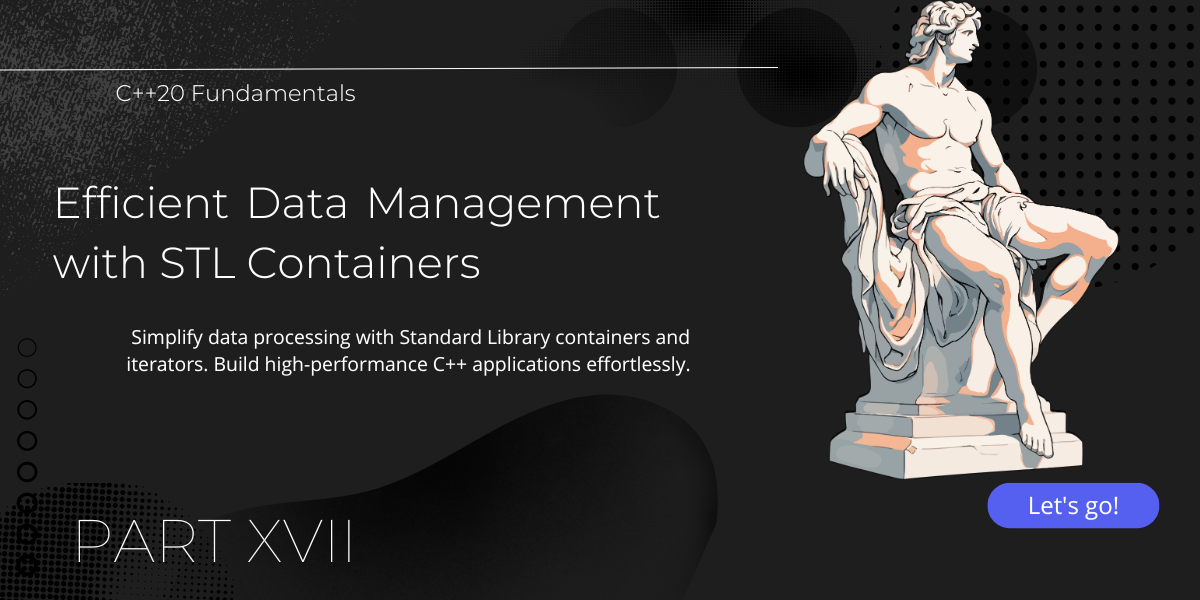
Course C++ Part 17: Standard Library Containers and Iterators
Course Description
This lesson teaches the use of Standard Library containers and iterators, providing you with tools to manage data efficiently and write cleaner code.
Master C++ Standard Library containers and iterators to write more efficient and scalable code! This course covers essential containers like vector, map, set, and unordered_map, helping you choose the right data structure for every task. You'll also explore iterators, from basic to advanced, learning how they enable seamless data traversal and manipulation. Understand the inner workings of container memory management, iterator invalidation, and performance optimization techniques. By the end of this course, you'll be able to write clean, maintainable, and high-performance C++ applications with confidenc
Course Curriculum
- 001. Lesson 17 Overview—Parallel Algorithms and Concurrency A High-Level View
- 002. Introduction
- 003. Standard Library Parallel Algorithms
- 004. Example Profiling Sequential and Parallel Sorting Algorithms
- 005. Example Profiling Sequential and Parallel Sorting Algorithms (Continued)
- 006. When to Use Parallel Algorithms
- 007. Execution Policies
- 008. Example Profiling Parallel and Vectorized Operations
- 009. Additional Parallel Algorithm Notes
- 010. Thread States and the Thread Life Cycle
- 011. Launching Tasks with stdjthread
- 012. Defining a Task to Perform in a Thread
- 013. Executing a Task in a stdjthread
- 014. How jthread Fixes thread
- 015. Producer–Consumer Relationship A First Attempt
- 016. Producer–Consumer Relationship A First Attempt—UnsynchronizedBuffer
- 017. Producer–Consumer Relationship A First Attempt—Main Application
- 018. Producer–Consumer Synchronizing Access to Shared Mutable Data
- 019. Class SynchronizedBuffer Mutexes, Locks and Condition Variables
- 020. Testing SynchronizedBuffer
- 021. Producer–Consumer Minimizing Waits with a Circular Buffer
- 022. Implementing a Circular Buffer
- 023. Testing Class CircularBuffer
- 024. Cooperatively Canceling jthreads
- 025. Launching Tasks with stdasync
- 026. A Brief Intro to Atomics
- 027. A Brief Intro to Atomics—Incrementing an int, a stdatomicint and a stdatomic refint from Two Concurrent Threads
- 028. Coordinating Threads with C++20 Latches and Barriers
- 029. C++20 stdlatch
- 030. C++20 stdlatch—Demonstrating Latches
- 031. C++20 stdbarrier
- 032. C++20 stdbarrier—Demonstrating a Barrier
- 033. C++20 Semaphores
- 034. C++20 Semaphores—Producer–Consumer Using C++20 stdbinary semaphores