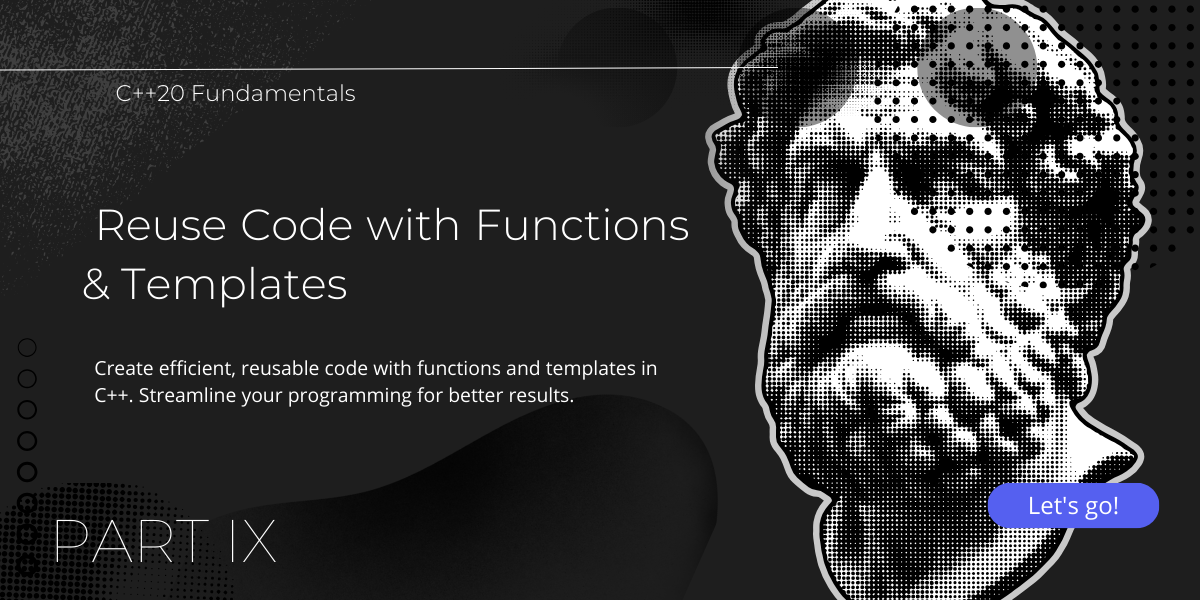
Course C++ Part 9: Functions and an Intro to Function Templates
Course Description
This lesson teaches you how to write functions in C++ and introduces function templates, helping you write more modular and reusable code.
Functions and an Intro to Function Templates explores the fundamentals of defining and using functions in C++. Learn about function parameters, return types, scope, overloading, and best practices for writing modular and efficient code. The course also introduces function templates, explaining how to create flexible and reusable functions that work with different data types. Through practical examples, gain a deeper understanding of structured programming and generic programming.
Course Curriculum
- 001. Lesson 9 Overview Custom Classes
- 002. Test-Driving an Account Object
- 003. Account Class with a Data Member
- 004. Account Class Custom Constructors
- 005. Software Engineering with Set and Get Member Functions
- 006. Account Class with a Balance
- 007. Time Class Case Study Separating Interface from Implementation
- 008. Class Scope and Accessing Class Members
- 009. Access Functions and Utility Functions
- 010. Time Class Case Study Constructors with Default Arguments, Part 1
- 011. Time Class Case Study A Subtle Trap--Returning a Reference or a Pointer to a private Data Member
- 012. Default Assignment Operator
- 013. const Objects and const Member Functions
- Composition Objects as Members of Classes
- friend functions and friend classes
- The this pointer--Implicitly and Explicitly Using the this Pointer to Access an Object's Data Members
- The this pointer--Using the this Pointer to Enable Cascaded Function Calls
- static Class Members--Classwide Data and Member Functions
- Aggregates and C++20 Designated Initializers
- Objects Natural Case Study Serialization with JSON and cereal--Introduction