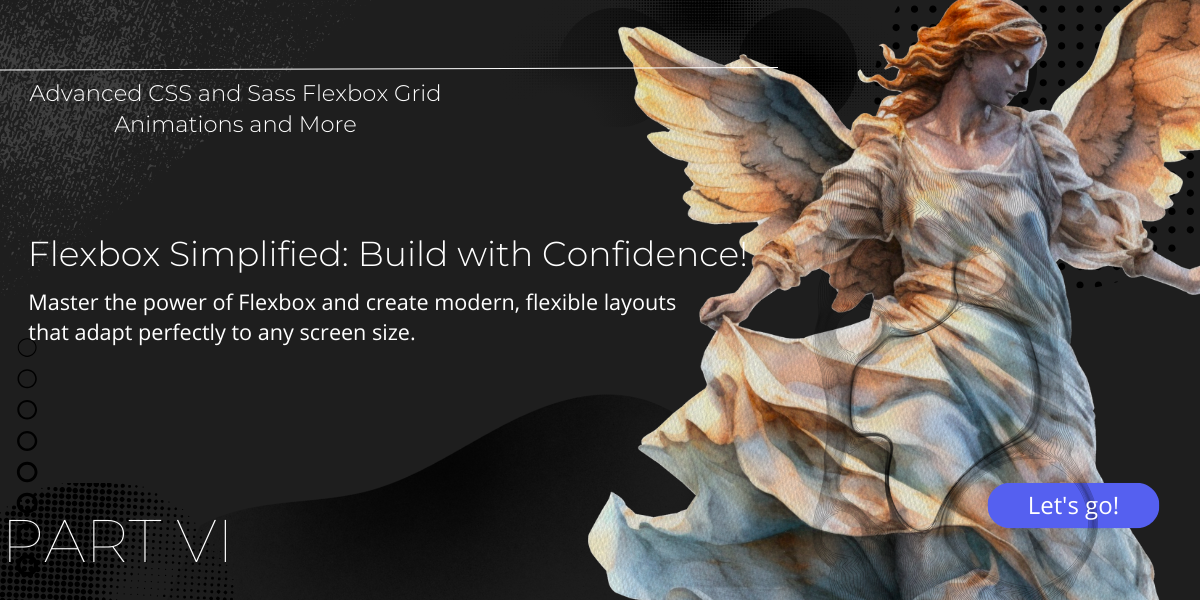
Course C++ Part 6: Intro to C++ Programming
Course Description
This introductory lesson covers the basics of C++ programming, providing you with the foundational knowledge needed to build more complex applications.
Start your journey into C++ programming with this beginner-friendly course! You'll learn the fundamental concepts of C++, including variables, data types, operators, control structures, and basic functions. We’ll guide you through writing, compiling, and running your first C++ programs, helping you develop a solid foundation in programming logic. Whether you’re completely new to coding or transitioning from another language, this course provides the essential knowledge to kickstart your C++ development skills with confidence.
Course Curriculum
- 001. Lesson 6 Overview arrays, vectors, Ranges and Functional-Style Programming
- 002. Initializing array Elements in a Loop
- 003. Initializing an array with an Initializer List
- 004. Range-Based for and C++20 Range-Based for with Initializer
- 005. Setting array Elements with Calculations; Introducing constexpr
- 006. Totaling array Elements with External Iteration
- 007. Using a Primitive Bar Chart to Display array Data Graphically
- 008. Using array Elements as Counters Reimplementing Lesson 5's Die Rolling Simulation
- 009. Using arrays to Summarize Survey Results
- 010. Sorting and Searching arrays
- 011. Multidimensional arrays
- 012. Intro to Functional-Style Programming
- 013. Intro to Functional-Style Programming What vs. How—Functional-Style Reduction with accumulate
- 014. Intro to Functional-Style Programming Passing Functions as Arguments to Other Functions—Introducing Lambda Expressions
- 015. Intro to Functional-Style Programming Filter, Map and Reduce—Intro to C++20’s Ranges Library
- 016. showValues Lambda for Displaying This Application’s Results
- 017. Generating a Sequential Range of Integers with viewsiota
- 018. Filtering Items with viewsfilter
- 019. Mapping Items with viewstransform
- 020. Combining Filtering and Mapping Operations into a Pipeline
- 021. Reducing a Range Pipeline with accumulate
- 022. Filtering and Mapping an Existing Container’s Elements
- 023. Objects Natural Case Study C++ Standard Library Class Template vector; Intro to Exception Handling