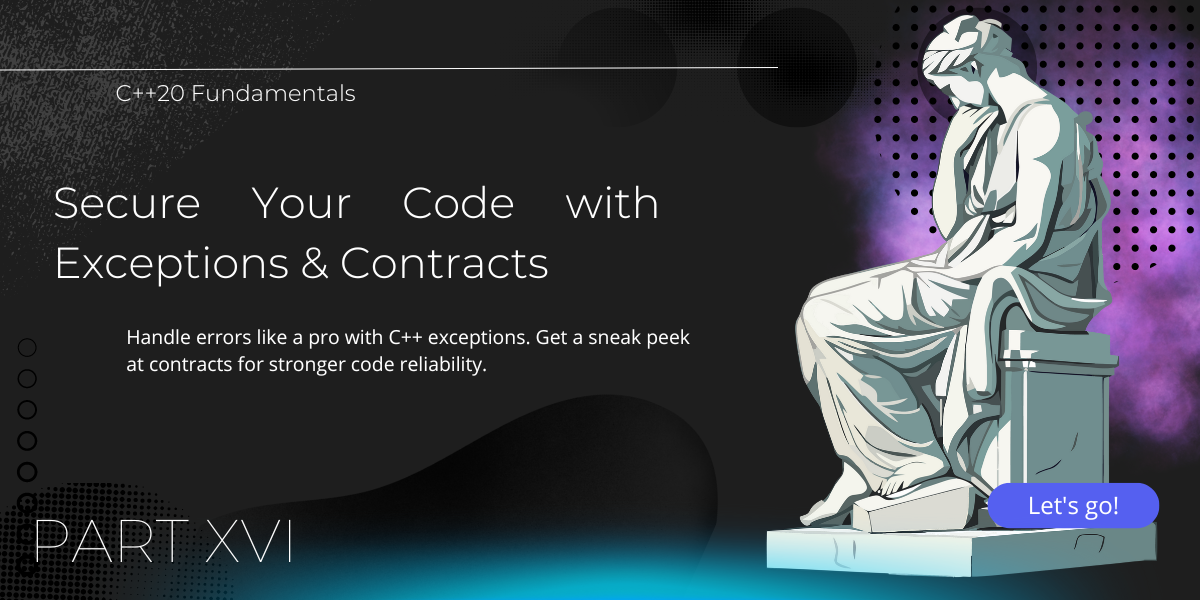
Course C++ Part 16: Exceptions and a Look Forward to Contracts
Course Description
This lesson covers exception handling in C++, teaching you how to manage errors effectively and introducing the concept of contracts for future-proofing your code.
Course Curriculum
- 001. Lesson 16 Overview—C++20 Modules Large-Scale Development
- 002. Introduction
- 003. Compilation and Linking Before C++20
- 004. Advantages and Goals of Modules
- 005. Example Transitioning to Modules—Header Units
- 006. Compiling with Header Units in Microsoft Visual Studio
- 007. Building a Pre-Release g++ 13 Docker Container
- 008. Compiling with Header Units in g++
- 009. Compiling with Header Units in clang++
- 010. Modules Can Reduce Translation Unit Sizes and Compilation Times
- 011. Example Creating and Using a Module
- 012. module Declaration for a Module Interface Unit
- 013. Exporting a Declaration
- 014. Exporting a Group of Declarations
- 015. Exporting a namespace
- 016. Exporting a namespace Member
- 017. Importing a Module to Use Its Exported Declarations
- 018. Importing a Module to Use Its Exported Declarations—Compiling This Example in g++
- 019. Importing a Module to Use Its Exported Declarations—Compiling This Example in clang++
- 020. Example Attempting to Access Non-Exported Module Contents
- 021. Example Attempting to Access Non-Exported Module Contents—g++ Error Messages
- 022. Example Attempting to Access Non-Exported Module Contents—clang++ Error Messages
- 023. Global Module Fragment
- 024. Separating Interface from Implementation—Example Module Implementation Units
- 025. Separating Interface from Implementation—Example Module Implementation Units (Compiling This Example in g++)
- 026. Separating Interface from Implementation—Example Module Implementation Units (Compiling This Example in clang++)
- 027. Separating Interface from Implementation—Example Modularizing a Class
- 028. Separating Interface from Implementation—Example Modularizing a Class (Compiling This Example in g++)
- 030. Separating Interface from Implementation—private Module Fragment
- 031. Partitions
- 032. Example Module Interface Partition Units
- 033. Example Module Interface Partition Units—Compiling This Example in g++
- 034. Example Module Interface Partition Units—Compiling This Example in clang++
- 035. Module Implementation Partition Units